섹션 6. 클래스
1강. 자바스크립트의 클래스 소개
1) allow.Js : ts 환경에서 js 파일 사용하도록 설정하기
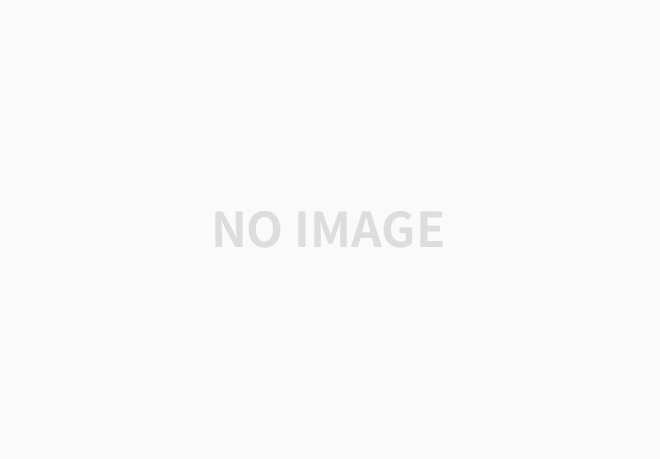
src폴더에 .js파일을 만들면 tscongfig에 오류가 난다.
"include" 옵션을 "src"로 설정했기 때문에 src 디렉토리 내의 모든 타입스크립트 파일을 타입스크립트 컴파일러가 관제하고 있다. 모니터링 중 ts 파일이 아닌 js 파일이 생겼기 때문에 오류가 나는 것이다.
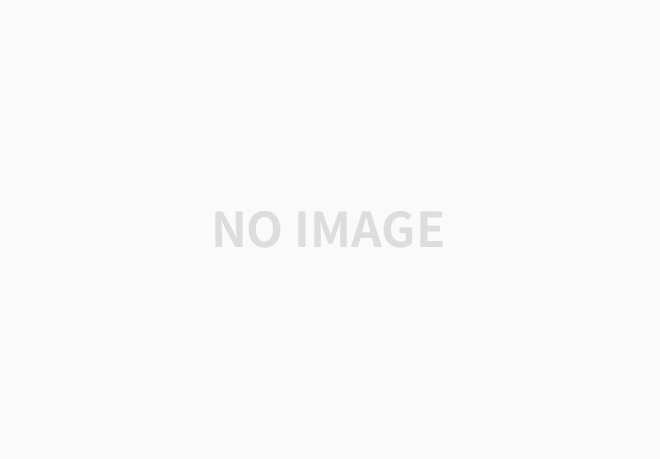
그래서 여기에 "allowJs": true를 추가한다.
그러면 오류가 사라진 것을 확인할 수 있다.
2) class
let studentA = {
name: "초보",
grade: "A+",
level: 5,
study() {
console.log("열심히 코딩함");
},
introduce() {
console.log("ㅎㅇㅎㅇ^O^~");
},
};
let studentB = {
name: "코더",
grade: "B+",
level: 3,
study() {
console.log("열심히는 코딩함");
},
introduce() {
console.log("ㅎㅇ=_=~");
},
};
위처럼 같은 학생을 여러번 쓸 경우, 코드중복이 발생한다.
데이터가 많아질수록 중복은 더 커질 것이고, 그로 인한 비효율이 매우 클 것이다.
이때, 클래스를 이용한다.
- 객체 : 붕어빵
- 클래스 : 붕어빵 틀(객체를 만들어내는 틀)
3) 생성자(constructor)
생성자 : 실제로 객체를 생성하는 역할을 하는 함수(메서드)
class Student { //표기법 : 파스칼 표기법(맨 첫 글자 대문자)
//아래를 필드라고 함 : 클래스가 만들어낼 객체의 프로퍼티, 어떤 붕어빵을 찍어낼지 정의하면 된다.
name;
grade;
level;
constructor(name, grade, level) { //매개변수로 받아주고, 실제로 만들 객체의 프로퍼티 값으로 설정
this.name = name;
this.grade = grade;
this.level = level;
}
}
this : 지금 클래스가 만들고 있는 객체
지금 생성하고 있는 객체 name(좌항) 프로퍼티의 값을 매개변수에 저장된 name(우항)으로 설정
이렇게 설정하면 이제 student를 공장처럼 찍어낼 수 있는 준비가 된 것
let studentB = new Student("코더", "A+", 3);
클래스를 호출해서 객체를 만들어줄 때는 new를 붙여줘야 한다.
new : 새로운 객체를 만들어라! 는 의미
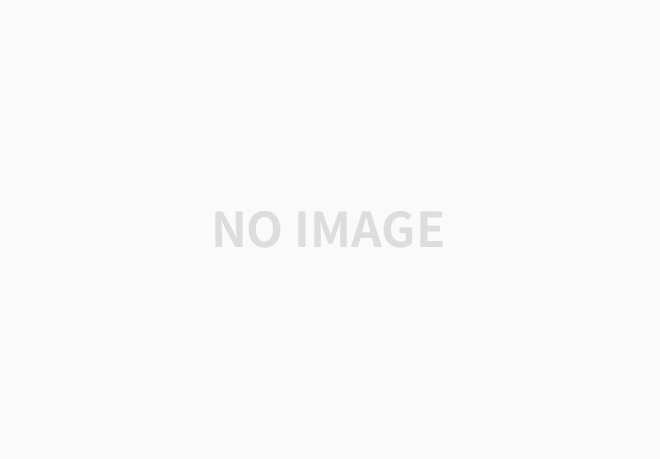
new + class 이름 + ( 인수 )
→ class에 있는 생성자 호출
4) 인스턴스(Instance)
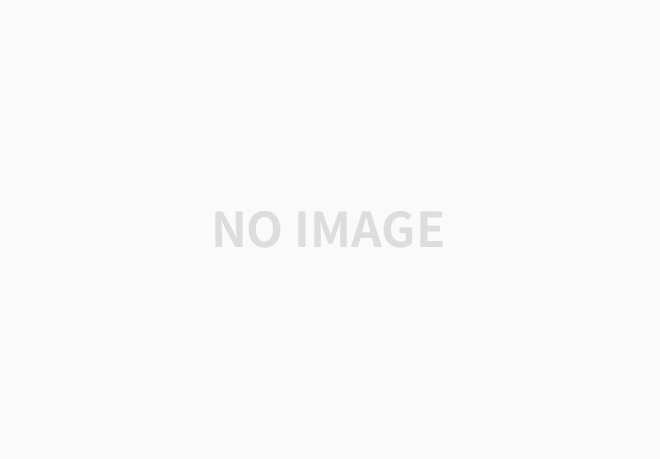
studentB라는 객체는 Student 클래스로 만든 객체고 프로퍼티로는 name, grade, level이 있다는 것이 잘 출력됨
인스턴스 : 클래스를 이용해서 만든 객체
즉, 위의 studentB는 student instance이다.
5) 메서드(Method)
class Student {
name;
grade;
level;
constructor(name, grade, level) {
this.name = name;
this.grade = grade;
this.level = level;
}
//메서드
study() {
console.log("열심히 코딩함");
}
introduce() {
console.log("ㅎㅇㅎㅇ^O^~");
}
}
let studentB = new Student("코더", "A+", 3);
console.log(studentB);
studentB.study();
studentB.introduce();
studentA에서 썼던 객체의 형태와 동일하게 클래스에 메서드를 추가한다.
그 뒤에는 study 메서드와 introduce 메서드를 모두 호출해서 쓸 수 있다.
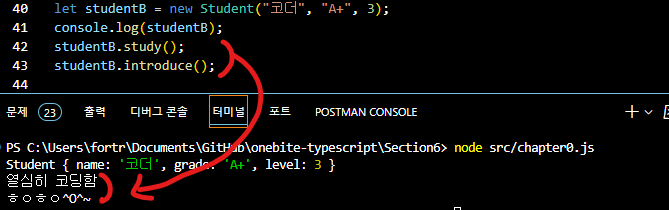
객체 매서드 : 각각이 프로퍼티로 취급 - 콤마, 쉼표 필요

하지만 클래스 안에서는 쉼표를 찍지 않는다.
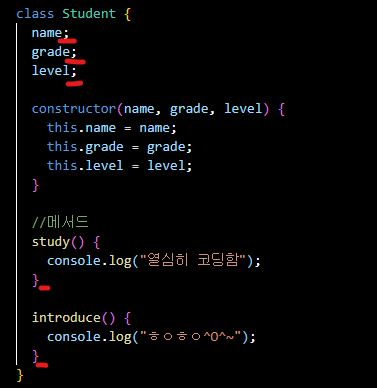
심지어 name, grade, level 뒤에도 쉼표가 아니라 ;가 쓰인다.
6) 변경되는 이름으로 호출하기
introduce() {
console.log(`ㅎㅇㅎㅇ~ ${this.name}입니다^O^~`);
}
}
`` : 템플릿 리터럴
${this.name}으로 넣으면 this.name에 따라 바뀐 이름이 출력된다.
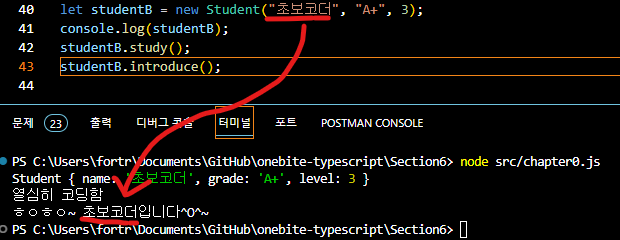
7) 프로퍼티 추가하기
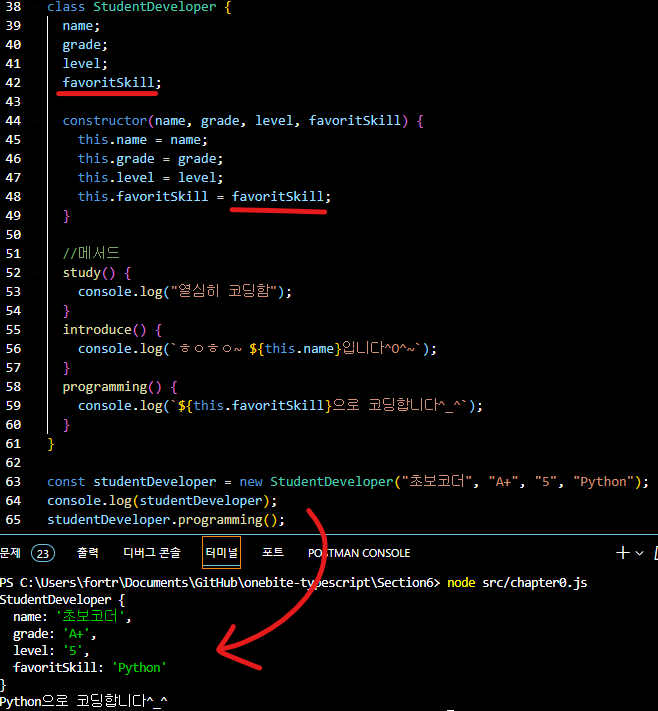
프로퍼티를 추가할 수도 있다.
8) 상속
보다보면 student와 class StudentDeveloper가 매우 중복된다.
이 경우, 상속(확장)을 사용하면 된다.
class Student {
name;
grade;
level;
constructor(name, grade, level) {
this.name = name;
this.grade = grade;
this.level = level;
}
//메서드
study() {
console.log("열심히 코딩함");
}
introduce() {
console.log(`ㅎㅇㅎㅇ~ ${this.name}입니다^O^~`);
}
}
class StudentDeveloper extends Student {
favoritSkill;
constructor(name, grade, level, favoritSkill) { //매개변수 name, grade, age는 지우면 안됨
super(name, grade, level); //인수 그대로 부모 클래스의 생성자 호출 : this.name = name 으로 설정되는 것
this.favoritSkill = favoritSkill;
}
//메서드
programming() {
console.log(`${this.favoritSkill}으로 코딩합니다^_^`);
}
}
- class StudentDeveloper의 field에서 name, grade, level 지움
- constructor에서 this.name, this.grade, this.level 지움
- 메서드에서 study(), introduce() 지움
- constructor에서 super(name, grade, level)로 부모 클래스의 생성자를 호출
중복되는 값을 지우는 것은 동일하나, constructor에서 super 함수 호출하여 인수를 전달하는 단계가 필요
'TypeScript' 카테고리의 다른 글
0062. TypeScript 공부하기11 - 클래스, 암묵적 Any 해결법, 접근제어자, 인터페이스와 클래스 (1) | 2024.02.11 |
---|---|
0060. TypeScript 공부하기9 - 인터페이스, 표기법, 확장, 선언 합치기, 모듈 보강 (1) | 2024.02.11 |
0059. TypeScript 공부하기8 - 함수 오버로딩, 사용자 정의 타입 가드(Custom Type Guard) (1) | 2024.02.10 |
0058. TypeScript 공부하기7 - 함수와 타입, 함수 타입 표현과 호출 시그니처, 호환성(반환값, 매개변수) (1) | 2024.02.10 |
0046. TypeScript 공부하기6 - 타입 좁히기, 서로소 유니온 타입 (1) | 2024.01.23 |